Did you know 92% of modern C++ developers use function overloading and overriding? These techniques help make their code more efficient and scalable. They are key to writing good C++ code.
In this guide, we’ll look at the differences between function overloading and overriding. You’ll learn how to use these important programming techniques. This article is for both new and experienced C++ developers. It will help you use polymorphism to its fullest in your projects.
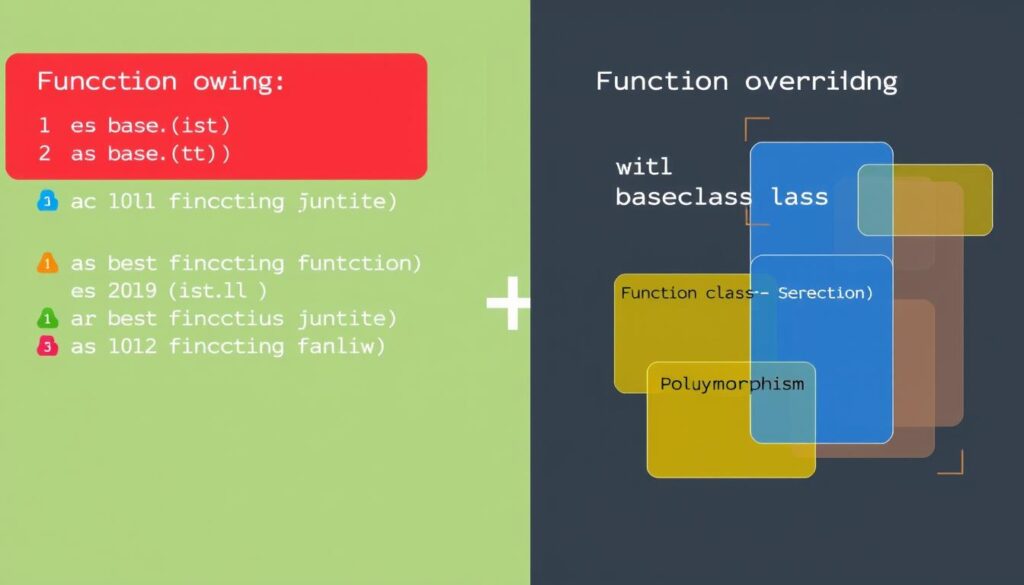
Key Takeaways
- Function overloading allows multiple functions with the same name but different parameters within a class.
- Function overriding involves redefining a function in a derived class with the same name and signature as the base class.
- Function overloading is a compile-time polymorphism technique, while function overriding is a runtime polymorphism mechanism.
- Function overloading does not require inheritance, while function overriding is dependent on inheritance.
- Understanding the nuances between function overloading and overriding is crucial for implementing effective object-oriented programming in C++.
Understanding Polymorphism in C++
Polymorphism is key in C++ that lets different types of objects act the same. It makes your code reusable and flexible. There are two main types: compile-time polymorphism and run-time polymorphism.
Types of Polymorphism
Compile-time polymorphism, or static polymorphism, uses function and operator overloading. It’s decided at compile time, when the right function or operator is picked based on the arguments.
Run-time polymorphism, or dynamic polymorphism, uses function overriding and virtual functions. The function to use is chosen at runtime, based on the object’s type.
Role in Modern C++ Programming
Polymorphism is vital in modern C++ for code reuse and better readability. It lets you write code that works with various types, reducing code duplication. This makes your code easier to maintain.
Basic Concepts and Terminology
To grasp polymorphism in C++, learn these key terms:
- Static Binding: Resolving function calls at compile-time, for compile-time polymorphism.
- Dynamic Binding: Resolving function calls at runtime, for run-time polymorphism.
- Overloading: Defining multiple functions with the same name but different parameters, for compile-time polymorphism.
- Overriding: Redefining a virtual function in a derived class, for run-time polymorphism.
Mastering these concepts will help you use polymorphism effectively in your C++ projects.
What is Function Overloading and How It Works
In C++, function overloading lets you have many functions with the same name but different parameters. This is called compile-time polymorphism. The compiler picks the right function to use based on the arguments given.
The rules for function overloading in C++ say that functions with the same name must differ in their parameters. This way, you can have different versions of a function for different situations. It makes your code easier to understand and more adaptable.
Here’s an example of function overloading in C++:
- You can make a function called
add()
that adds two integers. - Then, you can create another
add()
function for adding two floating-point numbers. - When you call
add()
, the compiler picks the right version based on the arguments.
This method makes your code easier to read, more flexible, and less repetitive. You don’t need to remember many function names for similar tasks.
Function overloading in C++ is a powerful tool. It lets you have many functions with the same name but different parameters. This improves your code’s readability, flexibility, and reduces repetition.
Function Overloading and Function Overriding in C++ with Example
In C++, function overloading and function overriding are key concepts. They help in making code flexible and easy to maintain. It’s important for developers to know the difference between these two.
Implementation Guidelines
Function overloading means having several functions with the same name but different parameters. The right function is chosen based on the arguments given. This choice is made at compile-time.
Function overriding, however, is when a child class changes a function from its parent class. This change is made at run-time, thanks to dynamic binding.
Common Use Cases
- Operator overloading lets you define how operators work with your own data types.
- Method overriding is used in object-oriented programming. It allows a child class to offer a unique version of a parent class’s method.
- Inheritance is a core C++ concept. It makes function overriding possible, letting a child class use and change its parent’s functions.
Best Practices
Function overloading is good for simple cases. It’s clear and easy to read. But for complex situations, function overriding is better. It’s especially useful in object-oriented programming.
Overloading | Overriding |
---|---|
Occurs at compile-time | Occurs at run-time |
Allows for the same function name with different parameters | Allows for the same function name with the same parameters |
Does not require inheritance | Requires inheritance |
Example: void print(int x); , void print(float x); | Example: Derived class overriding the drive() function of the base class Vehicle |
Deep Dive into Function Overriding
Function overriding is key in object-oriented programming (OOP). It lets a child class give its own version of a method from its parent class. This is called run-time polymorphism and is vital in C++.
In C++, if a child class overrides a parent class’s function, its version is used at runtime. So, calling the function on a child class object will run the child’s version, not the parent’s.
To override a function in C++, follow these steps:
- The child class’s function must have the same name, parameters, and return type as the parent’s.
- The child class function must be marked as
virtual
in the parent class for the right version to be called. - Using the
override
keyword in the child class function shows it’s overriding a virtual function from the parent, helping avoid errors.
Function overriding is great for reusing code, making programs more modular and specialized. It’s used a lot in polymorphism, where different classes can act like a common superclass. But, dealing with deep inheritance and many overridden functions can be complex and hard to maintain.
Language | Function Overriding Support |
---|---|
C++ | Supported using virtual functions and the override keyword |
Java | Supported as a core feature of the language |
Python | Supported due to the dynamic nature of the language |
C# | Supported using the override keyword |
JavaScript | Supported through prototype-based inheritance |
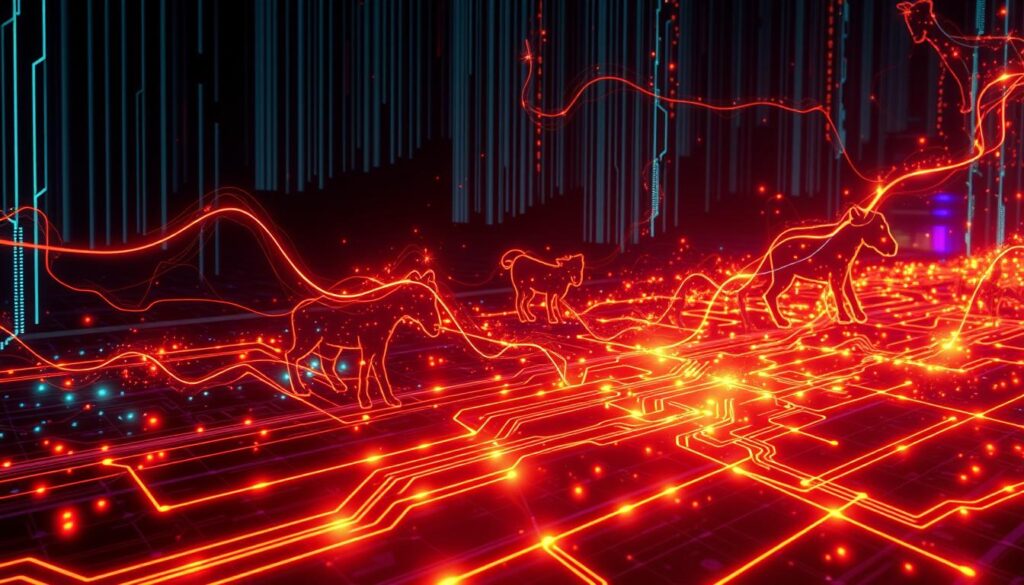
Virtual Functions and Dynamic Binding
Virtual functions in C++ are key for dynamic binding and runtime polymorphism. They let your program choose the right function to run at runtime. This choice depends on the object’s actual type. This flexibility is vital for making code that’s easy to extend and maintain, especially with inheritance and polymorphism.
Working with Virtual Functions
To make a virtual function, declare it as virtual in the base class. When a derived class overrides this function, the right version will run at runtime. This is called dynamic binding.
Runtime Polymorphism Implementation
Virtual functions are crucial for runtime polymorphism in C++. They ensure the right function is called, even if the variable is a base class pointer or reference. This makes your code more adaptable and efficient.
Memory Management Considerations
When using virtual functions, remember to manage memory well. If you delete a derived class object through a base class pointer, make sure the base class has a virtual destructor. This prevents memory leaks and ensures resources are released correctly.
In summary, virtual functions and dynamic binding are essential in C++. They help create flexible and adaptable code. By mastering these concepts, you can build powerful applications that meet changing needs and handle various object types well.
Key Differences Between Overloading and Overriding
As a C++ programmer, it’s key to know the difference between function overloading and overriding. These concepts are related but serve different roles. They have unique features you should grasp.
Compile-time Polymorphism vs. Runtime Polymorphism
- Function overloading uses compile-time polymorphism. The compiler picks the right function based on the arguments given.
- Function overriding, however, is about runtime polymorphism. The actual function to use is decided at runtime, based on the object type.
Inheritance and Function Signatures
- Overloaded functions can be in the same class, without needing inheritance. But, overridden functions must be in a child class. They must also have the same function signature as the parent class.
- Overloaded functions can have different parameters or numbers. But, overridden functions must match the parent class’s parameters and numbers exactly.
Performance and Flexibility
- Function overloading can make code more complex. It requires manual checks of parameters. But, it also offers more flexibility in defining functions.
- Function overriding is simpler. It fits better with object-oriented programming. It allows for dynamic dispatch and runtime polymorphism.
Knowing the differences between compile-time polymorphism (overloading) and runtime polymorphism (overriding) is vital. It helps you design and implement C++ applications better. This ensures they perform well and are easy to maintain.
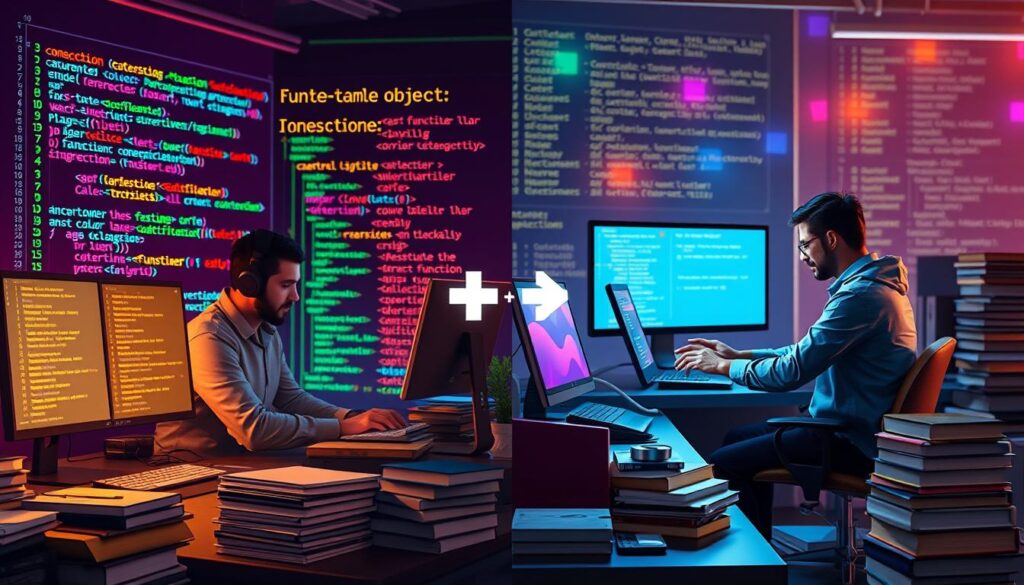
Common Pitfalls and How to Avoid Them
Exploring C++ function overloading and overriding can be tricky. Knowing common pitfalls helps you avoid them. This way, you can work with these programming concepts more smoothly.
Debugging Strategies
Debugging is a big challenge with function overloading and overriding. Here are some strategies to help:
- Make sure overloaded functions have different parameters to avoid confusion.
- Use the virtual keyword when you’re overriding to avoid issues.
- Be careful with function signatures to avoid hiding base class functions.
- Use your IDE’s debugger to find and fix problems step by step.
Performance Optimization Tips
Function overloading and overriding improve code readability but can affect performance. Here are tips to keep your C++ code running well:
- Try to reduce virtual function calls to avoid slowdowns.
- Don’t overdo function overloading to keep things simple and efficient.
- Manage memory well to avoid leaks with polymorphic objects.
- Use profiling tools to find and fix slow parts of your code.
Code Maintenance Considerations
Keeping C++ code that uses function overloading and overriding up to date needs careful planning. Here are some tips for maintaining your code:
Consideration | Best Practice |
---|---|
Function Naming | Choose names that are clear and descriptive to make your code easier to read. |
Documentation | Write detailed documentation for overloaded and overridden functions. Include what they do, what they take, and what they return. |
Consistency | Stick to a consistent method for function overloading and overriding. This makes your code easier to understand and maintain. |
By tackling common issues and using good strategies for debugging, performance optimization, and code maintenance, you can make the most of function overloading and overriding. This ensures your C++ projects are strong and easy to keep up with.
Conclusion
In the world of C++ programming, function overloading and overriding are key tools. They help you write efficient, easy-to-maintain, and flexible code. By learning these concepts, you can make the most of C++ and create complex applications.
Function overloading lets you have many functions with the same name but different parameters. This makes your code easier to read and use. It also makes your code more intuitive for users. Function overriding, on the other hand, lets you change functions from base classes in derived classes. This makes your C++ programs more adaptable and scalable.
As you keep learning C++ programming, always use object-oriented programming principles. This includes function overloading and overriding. Doing so will help you build strong, expandable, and user-friendly apps. These apps will meet the needs of people in India and worldwide.
FAQ
What is the difference between function overloading and function overriding in C++?
The main differences are: 1) Overloading happens without inheritance, but overriding needs it. 2) Overloading is figured out at compile-time, while overriding is at run-time. 3) Overloaded functions have different parameters, but overridden ones have the same. 4) Overloading is for compile-time polymorphism, and overriding is for run-time.
How does function overloading work in C++?
In C++, function overloading lets you have many functions with the same name but different parameters. The compiler picks the right version based on the arguments given. This makes code more intuitive and flexible.
What is function overriding in C++ and how is it implemented?
Function overriding in C++ happens when a child class has a function with the same name and signature as a parent class. This lets the child class offer a specific version of a function already in the parent class. It’s how the child class can do something different from the parent class.
What is the role of virtual functions in implementing runtime polymorphism in C++?
Virtual functions in C++ are key for runtime polymorphism. They let a program choose which function to call at runtime, based on the object’s actual type. This makes code more flexible and open to change, especially when dealing with inheritance and polymorphism.
What are some common pitfalls to be aware of when using function overloading and overriding in C++?
Common issues include unclear function calls in overloading, missing the ‘virtual’ keyword for overriding, and accidentally hiding base class functions. To steer clear of these, make sure overloading functions have clear parameter differences. Always mark functions for overriding as ‘virtual’ in the base class. And be careful with function signatures when overriding.
Also Read